Components and Aggregates
This chapter is about how to program with components and aggregates.
A component is one (or a number of) objects that handles a component in the plant. A
component can be for example a valve, a contactor, a temperature sensor or a frequency
converter. As these components are very common and exist in many different types of plants,
it is a great advantage if we can construct an object that contains all that is needed to
control and supervise the component, and is so general that it can be used in most
applications.
A component in ProviewR can be divided into a number of objects:
- a main object containing configuration data and data needed to supervise and operate
the component. It also contains the signal objects for the component.
- a function object that is placed in the plc program and that contains the code to
control the component.
- an I/O object that defines possible communication with for example a profibus module.
- a simulate object, used to test and simulate the system.
Furthermore an object graph, documentation, trends etc are included in the component.
An aggregate is a larger part in the plant than the component, and contains a number of
components. An aggregate can for example be a pump drive, consisting of the components
pump, motor, contactor and safety switch. In other respects, the aggregate is built as
a component with main object, function object, simulate object, object graph, documentation
etc.
Object orientation
ProviewR is an object oriented system, and components and aggregates are a field where
the benefits of object orientation are used. In the components, one can see how an object
is built by other objects, and that an attribute, besides from being a simple type as a float
or boolean, also can be an object, which in its turn is composed by other objects. An
attribute that is an object is called an attribute object. It is not quite analogous to
a free-standing object, as it lacks object head and an object identity, but apart from that
it contatins all the properties of a free-standing object in terms of methods, object graph
etc.
One example of an attribute object can be seen in the component object of a solenoid valve.
Here all the signal objects, two Di objects for limit switches, and a Do object for order,
are placed internally as attribute objects. Thus, we don't have to create these signals
separately. When the valve object is created, also the signals for the valve are created.
Another example of attribute objects is a motor aggregate that contains the component
objects for frequency converter, safety switch, motor etc. in the shape of attribute objects.
Another important property in object orientation is inheritance. With inheritance means that
you can create a subclass derived from an existing class, a superclass. The subclass
inherits all the properties of the superclass, but has also the possibility to extend
or modify some of the properties. One example is a component for a temperature sensor that
is a subclass to a general sensor object for analogous sensors. The only difference
between the temperature sensor class and its superclass is the object graph, where
the sensor value is presented in the shape of a thermometer instead of a bar. Another
example is a pump aggregate derived from a motor aggregate. The pump aggregate is
extended by a pump attribute object and also has a modified object graph that apart
from the motor control also displays a pump.
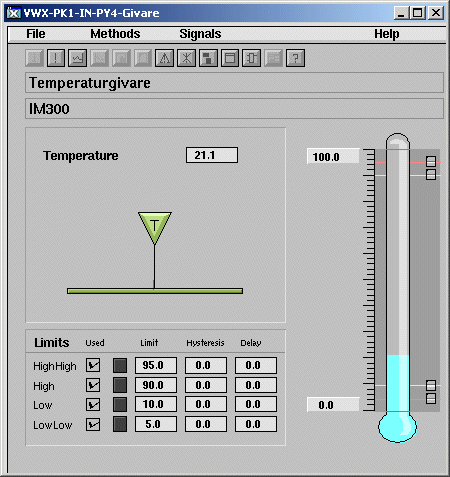
Fig Object graph for the class BaseTempSensor
Another property that we have introduced is the possibility to disable attributes. The
reason for this is that the component objects have to be as general as possible, to
be able to handle all variants of the plant component. A solenoid valve can, for example,
have a limit switch indicating valve open, but there are also solenoid valves with a
limit switch indicating valve closed, or valves with both switches or without switches.
Of course we could create four different component classes, one for each limit switch
alternative, but problems will arise when you start building aggregates of the components.
The number of variants of an aggregate will soon be unmanageable if you want to cover
all the variants of the components. If we, for example, want to create an aggregate
containing four solenoid valves, and there are four variants of each valve, there will
be 64 variants of the aggregate. If we want to build an aggregate containing four valve
aggregates the number of variants is 4096. The solution is to build a valve component
that contains both switches, but where you can disable one or both switches to be able
to handle all four limit switch variants. In this case attribute objects of class Di are
disabled, which means that they are not viewed in the navigator, ignored by the
I/O handling. Also the in code for the valve component and in the object graph this is taken
into consideration. The configuration is made in the configurator from the popup menu
where you under 'ConfigureComponent' can choose a configuration from the alternatives.
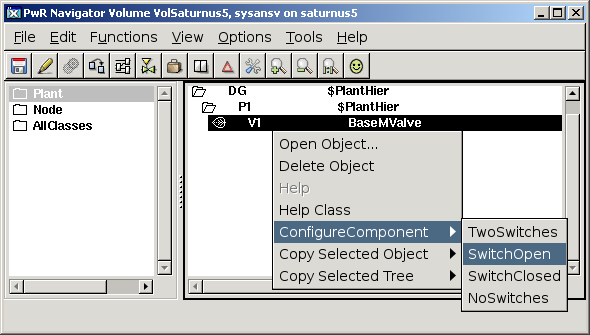
Fig The ConfigureComponent method for a BaseMValve.
Basecomponents
ProviewR contains a number of component and aggregate objects for common plant components,
e.g. temperature sensor, pressure sensor, pressure switch, solenoid valve, filter,
motor and fan drives. They are gathered in the classvolume BaseComponent. A basecomponent
can be used directly, and this is probably the usual way to use them, but the idea is also
that you from the basecomponents create libraries and classes for the specific components
you are using in your plant.
For solenoid valves there are the baseclass BaseMValve. If you have a solenoid valve
of type Durholt 100.103 you create a subclass with BaseMValve as superclass,
Durholt_Valve_100_103 and insert the configuration that is valid for this valve. You
also add a link to a datasheet and fill in the Specification attribute, which makes it
possible to identify and order spareparts to the valve. When using a Durholt_Valve_100_103
object you don't have to do so much configuring and adaptations because this is already
made in the class. In this way, component archives can be built for the types of component
you use in your plant.
A problem arises when you use aggregates. An aggregate contains basecomponents from
the BaseComponent volume, and if there are specific subclasses for a component, you
want to use these. The solution is the Cast function. A basecomponent in an aggregate
can be casted to a subclass, given that the subclass is not extended with new attributes.
The casting means that the component fetches initial values, configurations, methods,
object graph etc. from the subclass, i.e. in all situations it acts as the subclass it is
casted to. The casting is performed from the popup menu in the configurator, where you
in the 'Cast' alternative get a list of all the available subclasses. By selecting a
subclass the component is casted to this.
Pressure switch
Let's have a look at a relatively simple component, a pressure switch, to examine how
it is built and how to configure it. For pressure switches there is the base component
BasePressureSwitch, that is a subclass to BaseSupSwitch. As temperature, pressure and
limitswitches are quite alike, they have a common superclass. BaseSupSwitch has also a
superclass, Component, that is common for all component classes. The class dependency for
the pressure switch class can be written
Component-BaseSubSwitch-BasePressureSwitch
The Component class
Component contains the attributes Desciption, Specification, HelpTopic, DataSheet,
CircuitDiagram, Note and Photo that thus are present in all components. In Description
there is place for a short description, in Specification you enter the model specification,
the others are used to configure the corresponding methods in the operator environment.
BaseSubSwitch
From the superclass BaseSupSwitch the attributes Switch, AlarmStatus, AlarmText, Delay,
SupDisabled and PlcConnect are inherited.
- Switch is a Di object for the pressure switch. It should be connected to a channel
object in the node hierarchy.
- AlarmStatus shows the alarm status in runtime.
- AlarmText contains the alarm text for the alarm sent at alarm status. The alarm text
has the default value "Pressure switch, ", but can be changed to some other text.
Note that if the default text is kept, this will be translated if another language
is selected. If it is replaced by another text, the translation will fail.
- Delay is the alarm delay in seconds, default 0.
- SupDisabled indicates that the alarm is disabled.
- PlcConnect is a link to the function object in the plc code.
To the main object BaseSupSwitch there is a corresponding function object, BaseSupSwitchFo,
which also is inherited by the subclass BasePressureSwitch.
BasePressureSwitch
BasePressureSwitch doesn't have any attributes beyond those inherited from the superclasses.
The unique properties in BasePressureSwitch in the graphic symbol,
Components/BaseComponent/PressureSwitch, and the object graph where the switch symbol includes
a P for pressure.
Configuration
We open the configurator and create the main object, BasePressureSwitch, in the plant
hierarchy. In a suitable PlcPgm we insert a function object BaseSupSwitchFo and connect
it to the main object with the connect function. Select the main object and click with
Shift/Doubleclick MB1 on the function object. The function object contains the code for
the component, which for a BaseSubSwitch is an alarm that is sent when the switch signal
is lost.

Fig Main object with corresponding function object
The pressure switch is to be viewed in a Ge graph. We open the Ge graph and fetch the
subgraph BaseComponent/SupSwitch from the palette. The subgraph is adapted to a
BaseSubSwitch object, and all we have to do is to connect it to the main object. Select
the main object in the plant hierarchy and click with Shift/Doubleclick MB1 on the
subgraph.

Fig Graphic symbol, popup menu with methods and object graph
We have now accomplished a working component. We can of course also continue to configure
the method attributes with helptexts and links to photos, circuit diagrams and datasheet.
Control Valve
Let's have a closer look at a bit more complicated component, BaseCValve, that manoeuvers a
control valve. In contrast to the pressureswitch above, you also have to use
ConfigureComponent to configure the object, and there is also a simulate object that is
used to test the component.
Suppose now that we have a control valve, that is controlled by an analog output, and that gives
back the valve position in an analog input. Here we can use a BaseCValve. It has the analog
output 'Order' and the analog input 'Position', i.e. the signals we request. Apart from these,
there are two digital input signals for switch open and switch closed, but these can be
disabled by a configuration.
Configuration
We place the main object BaseCValve in the plant hierarchy and the function object BaseCValveFo
in a PlcPgm, and link them together with the Connect function. The functionobject has an
order input pin that we connect to a PID object. We also have to state that our valve does not
have any switches, and we do this by activating 'ConfigureComponent/PositionNoSwitches' in the
popupmenu for the main object. When we open the main object, and in this the Actuator object,
we shall find a Position signal, but no input signals for the switches.

Fig Main object with function object

Fig Configuring the main object with ConfigureComponent/PositionNoSwiches
In the HMI we place the subgraph BaseComponent/CValve in a Ge graph and connect it to the main
object.
We also want to be able to simulate the valve, to see that it works the way we want. For
simulation there is the function object BaseCValveSim that we place in a specific PlcPgm
for simulation, as this PlcPgm is not to be executed in the production system. The function
object is connected to the main object with the Connect function.
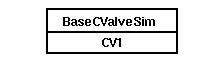
Fig Simulate object for the control valve
The configuration is finished and after building the simulation node we can test the system
and examine the result.
Pump drive
The next example is an aggregate, a pump drive with a frequency converter that communicates
via Profibus with the protocol PPO3. We will see how a component object in the aggregate,
in addition to the usual main object, function object and simulation object, also contains
I/O objects to fetch and send data via Profibus.
The class we use is BaseFcPPO3PumpAggr, and the class dependency for this class is
Aggregate-BaseFcPPO3MotorAggr-BaseFcPPO3PumpAggr
All aggregates have the superclass Aggregate that corresponds to the Component class for
components. The next superclass, BaseFcPPO3MotorAggr contains all the functionality for
the control. The pump class BaseFcPPO3PumpAggr extends the motor aggregate with a pump object
representing the mechanical pump, but this doesn't contain any signal or any additional
functionality. The pump aggregate also has its own object graph and graphical symbol.
Configuration
The main object BaseFcPPO3PumpAggr is placed in the plant hierarchy, and the function object,
that is inherited from the motor aggregate, BaseFcPPO3MotorAggrFo, is placed in a PlcPgm, and
they are linked together by the Connect function.
The main object has no less than 24 different configuration alternatives to choose between,
dependent on which of the components Fuse, Contactor, SafetySwitch, StartLock and CircuitBreaker
that are present in the construction. In our case we only have a contactor and a frequency converter
and we the choose the ConfigureComponent alternative CoFc.
Some components in an aggregate can have their own configurations. In this case the contactor
and the motor can be configured individually. Our contactor has two signals, a Do for order and
a Di for feedback, and this applies to the default configuration, i.e. we don't have to change
this. The motor, however has a temperature switch, and thus we select the motor component
and activate ConfigureComponent/TempSwitch in the popup menu.
The next step is to connect the signal objects to the channel objects. The contactor has a
Do for order and a Di for feedback that is to be connected to suitable channels in the node
hierarchy. The motor has a temperature switch in the shape of a Di that also should be
connected. The frequence converter contatins four signals, StatusWordSW (Ii), ActSpeed (Ai),
ControlWordCW (Io) and RefSpeed (Ao). These signals are exchanged with the frequency converter
via Profibus with the protocol PPO3. There is a specific Profibus module object for PPO3,
BaseVcPPO3PbModule, that contains the signals for PPO3 communication. The module is configured
by the Profibus configurator (see Guide to I/O System) and is connected to the
FrequencyConverter component in the pump aggregate. As the component object and module object
are adapted to each other, you don't have to connect each signal, you connect the component to
the module instead. By selecting the module object and activating ConnectIo in the popupmenu
for the FrequenceConverter component, the connection is made.
We also put the subgraph Component/BaseComponents/FccPPO3PumpAggr in an overview graph and
connect this to the main object. Furthermore we place the simulate object
BaseFcPPO3MotorAggrSim in specific simulate PlcPgm and connect it to the main object.
Mode
The pump aggregate contains a Mode object in which you configure where the pump is
controlled from. It can have the modes Auto, Manuel or Local which means:
- Auto: the pump is controlled by the plc program.
- Manual: the pump is controlled by the operator from the object graph.
- Local: the pump is controlled from a pulpet.
In the mode object you can configure the modes that apply to the pump in question.